This series of articles is my summary and harvest when learning webpack. This article is the third in a series of articles, including browser compatibility issues that are often overlooked by new front-end developers and the use of postcss
Contents
Browser Compatibility
There are many browsers in the current market, how should we solve the adaptation to different browsers?
The first thing to note is that browser adaptation here refers to support for different browser features: for example, CSS features, JavaScript syntax features, etc.
So, here is a question, how to determine which versions of browsers are supported?
In project development, we often see the following configurations in .broswerslistrc or package.json:
> 1% last 4 version not dead
Through these configurations, query the qualified browsers in the “Can I use” usage table , and then make feature compatibility for these browsers.
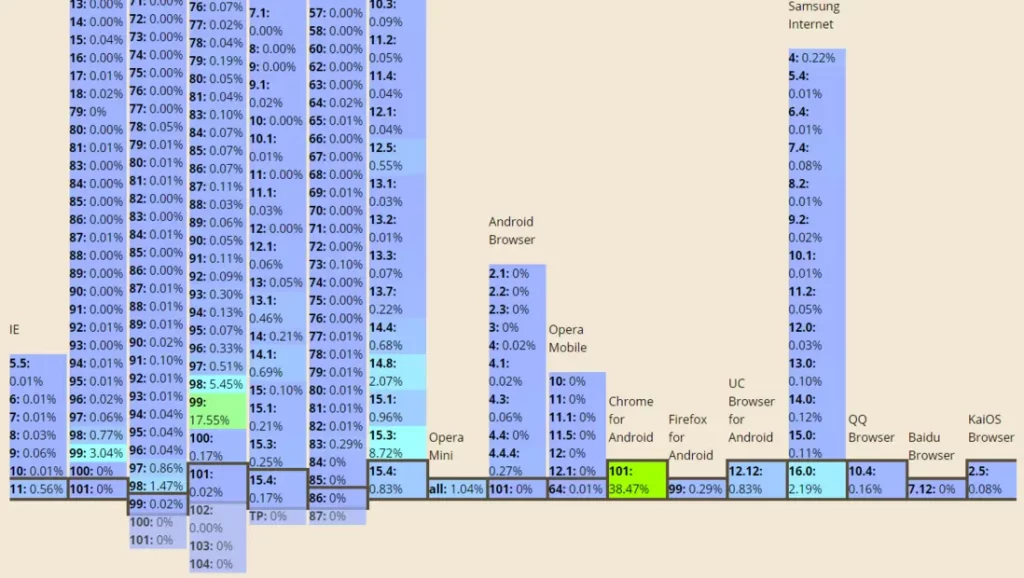
In order to be able to share these configurations among multiple front-end tools, a tool called browserlist needs to be used
Browserslist
Share the configuration of the target browser and Node.js version between different front-end tools. It is used for:
- Autoprefixer
- Babel
- postcss-preset-env
- eslint-plugin-compat
- stylelint-no-unsupported-browser-features
- postcss-normalize
- obsolete-webpack-plugin
browserslist writing rules
- defaults: Browserslist’s default browsers (>0.5%, last 2 versions, FIrefox ESR, not dead)
- 5%: Browser version selected through global usage statistics
- dead: No officially supported or updated browser for 24 months. Currently IE 10, IE_Mob 11
- last 2 versions: the latest 2 versions of each browser
- There are also some less commonly used configurations that can be viewed on the official website
The relationship between multiple conditions is shown in the following figure:
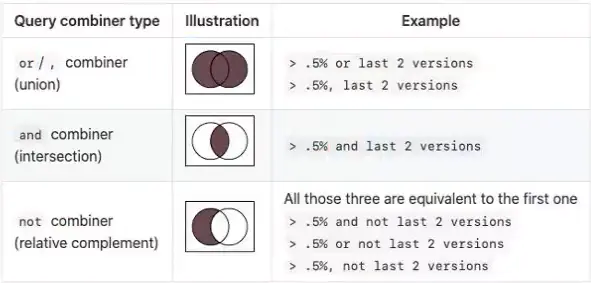
Instructions
- Add browserslist field in package.josn
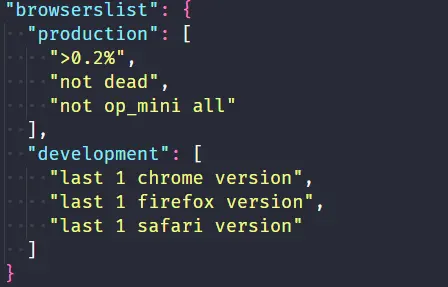
- Add the .browserslistrc file in the root directory and write the configuration in this file
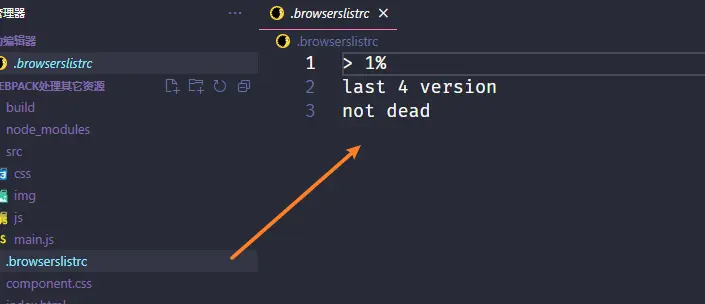
- command line usage
npx browserslist ">1%, last 2 versions, not dead"
npx browserslist
, you can also use it without adding parameters, if you don’t add parameters, it will go to the current directory to find.browserslistrc
conditions in the file
The above three methods of use, the first two are the methods we often use in our daily development. For example, autoprefixer and babel will automatically look for the browserslist field in package.json or the .browserslistrc file, and then automatically use the caniuse-lite tool to query eligible browsers, and then perform feature compatibility and other processing on the target browser.
caniuse-lite
The tool doesn’t send requests to Can I use… Support tables for HTML5, CSS3, etc , and then get eligible browser data. caniuse-lite itself is a small dataset that holds important parts of the data in a compact format.
Therefore, whether it is autoprefixer or babel, it only needs to filter out the corresponding browsers according to the .browserslistrc file
PostCSS
PostCSS is a tool that transforms styles through JavaScript, which can help us with CSS transformation and adaptation, such as automatically adding browser prefixes and resetting CSS styles. However, in order to achieve the above functions, the corresponding plug-ins must be used.
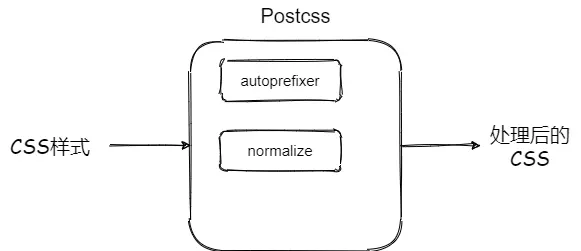
To use Postcss in webpack, you need to install postcss-loader and postcss first. Use postcss through postcss-loader, for specific functions, you need to install specific plugins
Instructions
- Write configuration directly in module module.exports = { module: { rules: [ { test: /\.css$/, exclude: /node_modules/, use: [ { loader: ‘style-loader’, }, { loader: ‘css-loader’, options: { importLoaders: 1, } }, { loader: ‘postcss-loader’, options: { postcssOptions: { plugins: [ require(‘autoprefixer’), // 添加浏览器前缀 require(‘postcss-preset-env’) // 该插件可以使用一些未来的CSS特性 ] } } } ] } ] } }
- Create a postcss.config.js file and add the loader to use in this file. This method is more concise than the first module.exports = { plugins: [ require(‘autoprefixer’), require(‘postcss-preset-env’) ] }
importLoaders
This option is used to process a CSS file and import another CSS file by @import
/* index.css */ @import "./test.css/" /* test.css */ :fullscreen { } .content { user-select: none; }
In this case, if the importLoaders option is not added, the styles in test.css will not be processed.
The reason is that when matching a css file, first use postcss-loader to process the index.css file, it will not process the imported test.css file according to the @import syntax. Then use css-loader for processing, css-loader can process the imported test.css file according to @import, which leads to the fact that the files in test.css are not processed by postcss.
In order to solve this problem, you can add an importLoaders option to the options of css-loader
{ loader: 'css-loader', options: { importLoaders: 1 // 该数字代表需要前面几个loader再次处理 } }
It means that when using css-loader, use several loaders before css-loader to process it once