by emanjusaka from
https://www.emanjusaka.top/2023/12/java-arrayList What can you do if the flowers on the other side bloom?This article welcomes sharing and aggregation. Please leave the original address for full text reprinting.
ArrayList is a Java class implemented using the List interface. As the name suggests, Java ArrayList provides the functionality of dynamic arrays, where the size of the array is not fixed. It implements all optional list operations and allows all elements, including null.
ArrayList
Inherited from AbstractList
, implements List
, RandomAccess
, Cloneable
, java.io.Serializable
these interfaces.
public class ArrayList <E> extends AbstractList <E> implements List <E>, RandomAccess, Cloneable, java.io.Serializable{ }
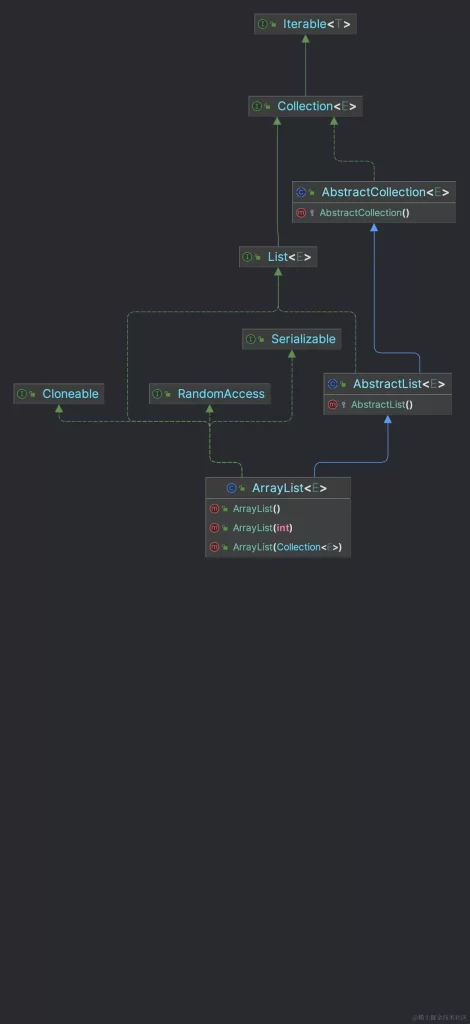
Contents
Constructor in ArrayList
//Default initialization capacity private static final int DEFAULT_CAPACITY = 10 ; // Shared empty array instance for empty instance. private static final Object[] EMPTY_ELEMENTDATA = {}; // Shared empty array instance for default size empty instance. We differentiate this from EMPTY_ELEMENTDATA to know how much to inflate when adding the first element. private static final Object[] DEFAULTCAPACITY_EMPTY_ELEMENTDATA = {}; public ArrayList () { this .elementData = DEFAULTCAPACITY_EMPTY_ELEMENTDATA; } public ArrayList ( int initialCapacity) { if (initialCapacity > 0 ) { this .elementData = new Object [initialCapacity]; } else if (initialCapacity == 0 ) { this .elementData = EMPTY_ELEMENTDATA; } else { throw new IllegalArgumentException ( "Illegal Capacity: " + initialCapacity); } } public ArrayList (Collection<? extends E> c) { Object[] a = c.toArray(); if ((size = a.length) != 0 ) { if (c.getClass() == ArrayList.class) { elementData = a; } else { elementData = Arrays.copyOf(a, size, Object[].class); } } else { // replace with empty array. elementData = EMPTY_ELEMENTDATA; } }
- Default constructor, constructs an empty list with an initial capacity of 10 (no parameter construction)
- Constructor with initial capacity parameters. (User-specified capacity)
- Constructs a list containing the elements of the specified collection, which are returned in order using the collection’s iterator
Automatic expansion
public void ensureCapacity ( int minCapacity) { int minExpand = (elementData != DEFAULTCAPACITY_EMPTY_ELEMENTDATA) // any size if not default element table ? 0 // larger than default for default empty table. It's already // supposed to be at default size. : DEFAULT_CAPACITY; //The minimum capacity is greater than the initial capacity if (minCapacity > minExpand) { ensureExplicitCapacity(minCapacity); } } private static int calculateCapacity (Object[] elementData, int minCapacity) { if (elementData == DEFAULTCAPACITY_EMPTY_ELEMENTDATA) { return Math.max(DEFAULT_CAPACITY, minCapacity); } return minCapacity; } private void ensureCapacityInternal ( int minCapacity) { ensureExplicitCapacity(calculateCapacity(elementData, minCapacity)); } private void ensureExplicitCapacity ( int minCapacity) { modCount++; // overflow-conscious code if (minCapacity - elementData.length > 0 ) // expansion grow(minCapacity); } private void grow ( int minCapacity) { // overflow-conscious code int oldCapacity = elementData.length; int newCapacity = oldCapacity + (oldCapacity >> 1 ); if (newCapacity - minCapacity < 0 ) newCapacity = minCapacity; if (newCapacity - MAX_ARRAY_SIZE > 0 ) newCapacity = hugeCapacity(minCapacity); // minCapacity is usually close to size, so this is a win: elementData = Arrays.copyOf(elementData, newCapacity); } private static int hugeCapacity ( int minCapacity) { if (minCapacity < 0 ) // overflow throw new OutOfMemoryError (); return (minCapacity > MAX_ARRAY_SIZE) ? Integer.MAX_VALUE : MAX_ARRAY_SIZE; }
When we create ArrayList in Java, its default capacity is 10. The ArrayList can automatically resize if more elements are added than the initial capacity. If you are sure of the minimum number of elements that need to be added to the ArrayList, you can specify it using the ensureCapacity() method.
- Initial capacity: When a new ArrayList is created, it is assigned an initial capacity. This initial capacity determines how many elements the ArrayList can accommodate without having to expand.
- Expansion factor: ArrayList also has an expansion factor, which determines how much the ArrayList’s capacity will increase when it needs to be expanded. The expansion factor is usually a number greater than 1, such as 1.5 or 2.
- Expansion condition: When ArrayList needs to add a new element, it checks whether its current capacity is sufficient. If the current capacity is not enough, it will be expanded.
- Expansion process: The expansion process includes the following steps:
- Creates a new array whose capacity is the current capacity multiplied by the expansion factor.
- Copies all elements in the ArrayList to a new array.
- Set the new array to the backing array of the ArrayList.
- Update the capacity of ArrayList to the new capacity.
Important features of ArrayList
- ArrayList inherits the AbstractList class and implements the List interface.
- ArrayList is initialized by size. However, if the collection grows, the size automatically increases; if an object is removed from the collection, the size automatically decreases.
- ArrayList allows us to access the list randomly.
- ArrayList cannot be used with primitive types such as int, char, etc. We need a wrapper class to handle this situation.
- ArrayList in Java can be regarded as vector in C++.
- ArrayList is out of sync. Its equivalent synchronization class in Java is Vector.
Methods in ArrayList
- add(int index, Object element): This method is used to insert a specific element at a specific position index in the list.
- add(Object o): This method is used to append a specific element to the end of the list.
- addAll(Collection c): This method is used to append all the elements in a specific collection to the end of the mentioned list, in the order in which the iterator of the specified collection returns the value.
- addAll(int index, Collection c): Used to insert all elements in a specific collection starting from the specified position into the mentioned list.
- clear(): This method is used to remove all elements from any list.
- clone(): This method is used to return a shallow copy of ArrayList in Java.
- contains(Object o): Returns true if this list contains the specified element.
- ensureCapacity(int minCapacity): If necessary, increase the capacity of this ArrayList instance to ensure that it can hold at least the number of elements specified by the minimum capacity parameter.
- forEach(Consumer<? super E> action): Perform the given operation on each element of Iterable until all elements are processed or the operation throws an exception.
- get(int index): Returns the element at the specified position in this list.
- indexOf(Object o): Returns the index of the first occurrence of a specific element, or -1 if the element is not in the list.
- isEmpty(): Returns true if this list does not contain any elements.
- lastIndexOf(Object o): Returns the index of the last occurrence of a specific element, or -1 if the element is not in the list.
- listIterator(): Returns a list iterator of the elements in this list (in correct order).
- listIterator(int index): Returns a list iterator of the elements in the list (in correct order), starting at the specified position in the list.
- remove(int index): Remove the element at the specified position in this list.
- remove(Object o): Removes the first occurrence of the specified element from this list (if it exists).
- removeAll(Collection c): Removes from this list all elements contained in the specified collection.
- removeIf(Predicate filter): Removes all elements from this collection that satisfy the given predicate.
- removeRange(int fromIndex, int toIndex): Removes from this list all elements with indexes between fromIndex (inclusive) and toIndex (exclusive).
- retainAll(Collection<?> c): Only retain elements contained in the specified collection in this list.
- set(int index, E element): Replace the element at the specified position in this list with the specified element.
- size(): Returns the number of elements in this list.
- spliterator(): Creates a late-bound and fail-fast Spliterator on the elements in this list.
- subList(int fromIndex, int toIndex): Returns a view of the portion of this list between the specified fromIndex (inclusive) and toIndex (exclusive).
- toArray(): This method is used to return an array containing all the elements of the list in the correct order.
- toArray(Object[] o): It is also used to return an array containing all the elements in this list in the same order as the previous method.
- trimToSize(): This method is used to trim the capacity of an ArrayList instance to the current size of the list.
Add elements to ArrayList
You can use add()
methods to add elements to an ArrayList.
// This will add "emanjusaka" to the end of the list aList.add( "emanjusaka" ); // This will add "emanjusaka" at the fourth position aList.add( 3 , "emanjusaka" );
Change elements in ArrayList
You can use set()
methods to change elements in an ArrayList. The index and new element are required, then this method updates the element present at the given index with the new given element.
package top.emanjusaka; import java.util.ArrayList; public class Main { public static void main (String[] args) { ArrayList<String> aList = new ArrayList <>(); aList.add( "emanjusaka" ); aList.add( "Tom" ); aList.add( "Jack" ); aList.set( 1 , "Jerry" ); System.out.println(aList); } }
Output result:
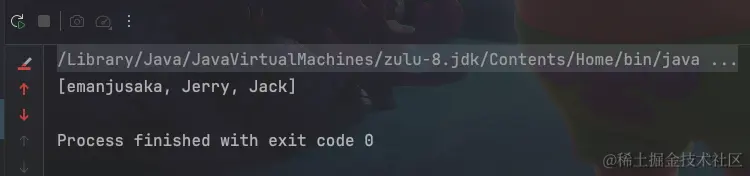
Remove elements from ArrayList
You can use remove()
methods to remove elements from an ArrayList.
package top.emanjusaka; import java.util.ArrayList; public class Main { public static void main (String[] args) { ArrayList<String> aList = new ArrayList <>(); aList.add( "emanjusaka" ); aList.add( "Tom" ); aList.add( "Jack" ); System.out.println(aList); aList.remove( "Tom" ); System.out.println(aList); aList.remove( 1 ); System.out.println(aList); } }
Output result:
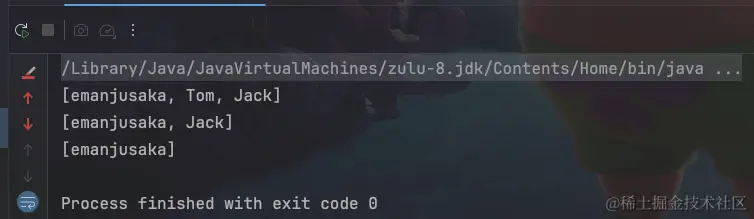
IterateArrayList
package top.emanjusaka; import java.util.ArrayList; public class Main { public static void main (String[] args) { ArrayList<String> aList = new ArrayList <>(); aList.add( "emanjusaka" ); aList.add( "Tom" ); aList.add( "Jack" ); for (String s : aList) { System.out.println(s); } } }
Output result:
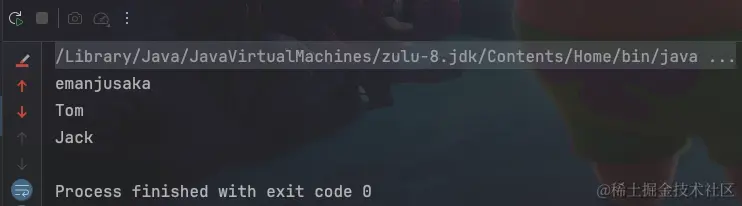
ArrayList size
You can use ArrayList’s size()
method to find the number of elements in an ArrayList.
package top.emanjusaka; import java.util.ArrayList; public class Main { public static void main (String[] args) { ArrayList<String> aList = new ArrayList <>(); aList.add( "emanjusaka" ); aList.add( "Tom" ); aList.add( "Jack" ); System.out.println( "Number of elements in ArrayList: " + aList.size()); } }
Output result:
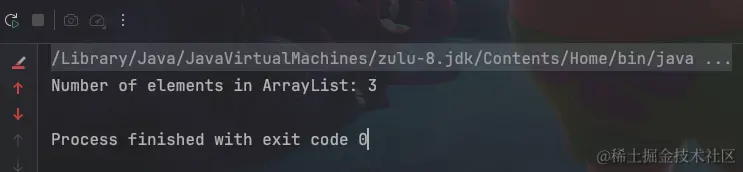
Sort ArrayList
sort()
You can sort an ArrayList using methods of the Collections utility class .
package top.emanjusaka; import java.util.ArrayList; import java.util.Collections; public class Main { public static void main (String[] args) { ArrayList<String> aList = new ArrayList <>(); aList.add( "emanjusaka" ); aList.add( "Tom" ); aList.add( "Jack" ); Collections.sort(aList); for (String s : aList) { System.out.println(s); } } }
Output result:
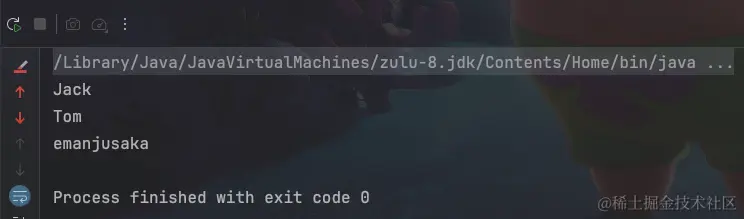
References
- jdk8 source code
- https://beginnersbook.com/2013/12/java-arraylist